What is the ServiceTimerProvider?
The ServiceTimerProvider API can be used by features to set feature specific timers and receive TimerEvents, when the timers fire.
![]() |
ServiceTimerProvider is currently only available in the Sentinel SIP frontend, but the concept eventually will be extended to the other cores. |
![]() |
The full ServiceTimerProvider API is available here. |
Accessing the ServiceTimerProvider
A feature gets a reference to a ServiceTimerProvider
as a resource adaptor provider object with the well known name "sentinel/servicetimerprovider"
. Your feature should implement InjectResourceAdaptorProvider
as shown in the example below.
@SentinelFeature(
featureName = MyFeature.NAME,
...
raProviderJndiNames = "sentinel/servicetimerprovider"
)
...
public class MyFeature
extends BaseFeature<..., ...>
implements InjectResourceAdaptorProvider
{
// ...
@Override
public void injectResourceAdaptorProvider(Object provider) {
if(provider instanceof ServiceTimerProvider){
this.serviceTimerProvider = (ServiceTimerProvider)provider;
}
}
private ServiceTimerProvider serviceTimerProvider;
public static final String NAME = "MyFeature";
}
Processing Service Timer Events
The purpose of the ServiceTimerProvider
is to provide features with a mechanism to be triggered at a scheduled time in the future via a TimerEvent
. Service Timer Events may be processed by:
In general, features should not attempt to perform their own Timer management using the SLEE Timer Facility. Instead, they should use the ServiceTimerProvider
to perform all timer operations.
Processing a Service Timer Event by Directly Invoking a Feature
If you want Sentinel to directly invoke a particular feature when your timer fires, use the setTimer
operations of the SentinelTimerProvider
interface that take a String
feature name as the first argument. For example:
// set a timer to expire in 5000ms, and cause a feature to be invoked.
long nowT = System.currentTimeMillis();
long expireT = nowT + 5000; // expire in 5000ms from now
TimerID timer = timerProvider.setTimer(NAME, // invoke me when the timer fires
expireT); // when to expire
![]() |
You may also register an existing Timer with the |
In the following diagram, a feature MyFeature
sets a timer using the ServiceTimerProvider
. The ServiceTimerProvider
remembers that MyFeature
should be triggered when the timer expires and uses the SLEE timer facility to set the timer. Finally the feature tells Sentinel it is done via featureHasFinished()
.
At some point in the future the timer expires. The SLEE triggers Sentinel in a new SLEE transaction. Sentinel defers to the ServiceTimerProvider
to find the name of a feature to invoke, and then directly invokes it by calling startFeature()
with the TimerEvent
as the trigger.
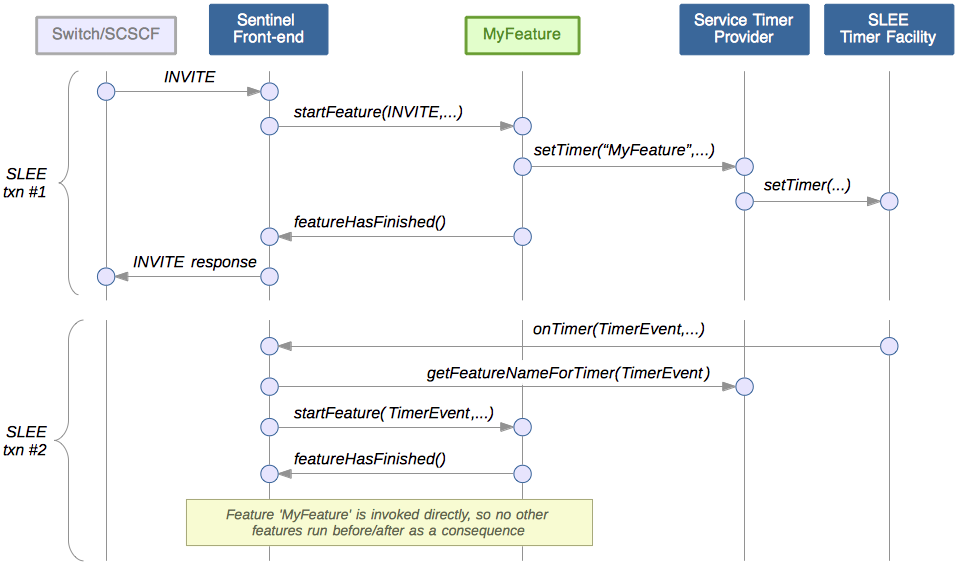
![]() |
A feature may set several timers. A feature may include the name of a different feature to be invoked. |
Processing a Service Timer Event by Invoking a Feature Execution Script
If you want Sentinel to find, and run, a feature execution script when your timer fires, use the setTimer
operations of the SentinelTimerProvider
interface that do not take a feature name argument. For example:
// set a timer to expire in 5000ms, and cause a feature execution script to be run.
long nowT = System.currentTimeMillis();
long expireT = nowT + 5000; // expire in 5000ms from now
TimerID timer = timerProvider.setTimer(expireT); // when to expire
![]() |
You may also register an existing Timer with the |
In the following diagram, a feature MyFeature
sets a timer using the ServiceTimerProvider
. The ServiceTimerProvider
uses the SLEE timer facility to set the timer. Finally the feature tells Sentinel it is done via featureHasFinished()
.
At some point in the future the timer expires. The SLEE triggers Sentinel in a new SLEE transaction. Sentinel searches for a feature execution script to run at the SipAccess_ServiceTimer
feature script execution point using the current value of the SentinelSelectionKey
. If a script is found, then Sentinel will run it.
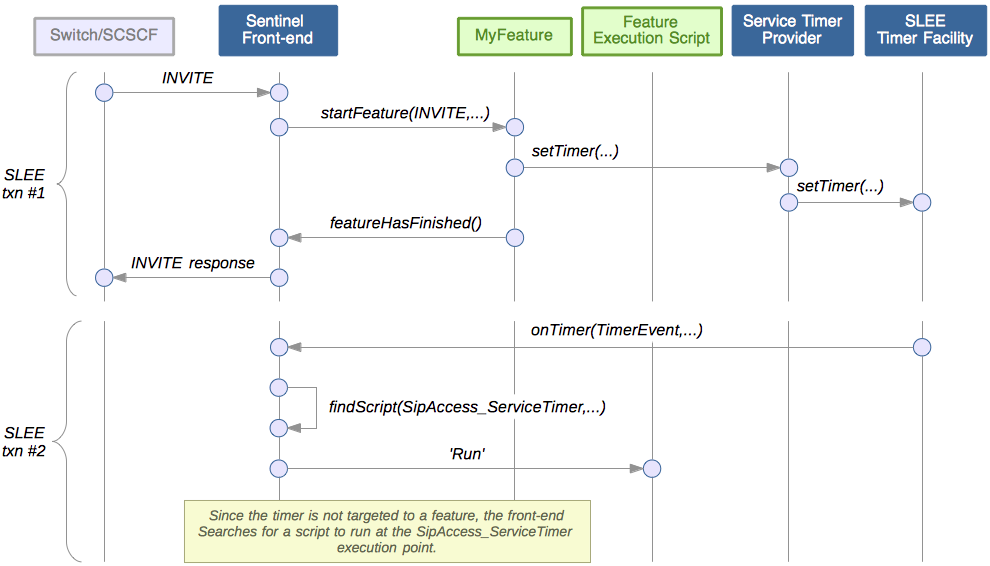
![]() |
See section Sentinel Selection Key for more information about the sentinel selection key. See section Feature Execution Scripts for more information about feature execution scripts. |
Cancelling a Timer
You cancel a timer that has been registered with the ServiceTimerProvider
with the cancelTimer
operation. For example:
// get the TimerID from session state
final TimerID timerID = sessionState.getNoResponseTimerID();
if (timerID != null) {
if (facilities.getTracer().isFinestEnabled())
facilities.getTracer().finest("Cancelling NoResponse timer: " + timerID);
// if we have a timer, then cancel it
timerProvider.cancelTimer(timerID);
}
Testing if a Timer is Set
You can check if a timer is currently set and registered with the provider with the timerIsSet
operation. For example:
final TimerID timerID = sessionState.getNoResponseTimerID();
if (timerProvider.timerIsSet(timerID)) {
// cancel the timer
timerProvider.cancelTimer(timerID);
}