The following is an example SBB that uses an sbbpart written using the generated sbbpart super class for the Ping Pong API.
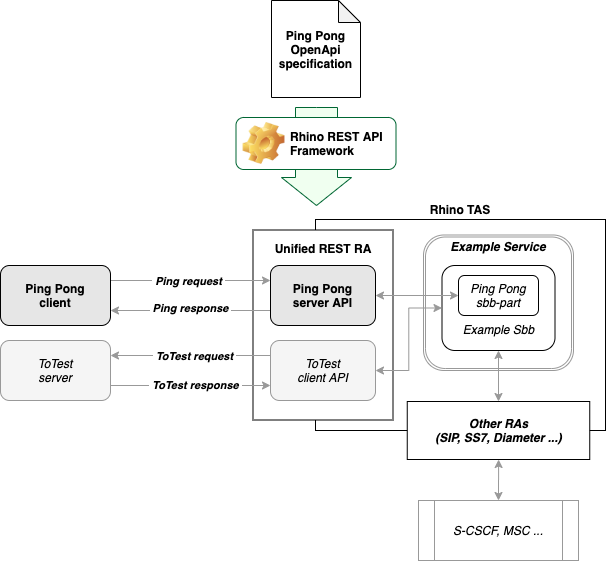
There are two aspects to this example:
-
A root SBB that defines an SBB local interface.
-
An sbb-part that extends the generated sbb-part superclass. The sbb-part will interact with the root SBB via the root SBB local interface.
![]() |
Advantages of using the generated sbb-part superclass
|
![]() |
Learn about Rhino TAS - Telecom Application Server and the JAIN SLEE (JSR 240). |
Create an SBB class and SBB local interface
SBB local interface
The sbb-part will interact with the root SBB by invoking methods on its SBB local interface.
In this example, the root SBB local interface includes a single operation triggerScenario()
.
public interface TriggerScenario {
boolean triggerScenario(String api, String scenario);
}
@Library(
id = @ComponentId(name = "@component.name@",
vendor = "@component.vendor@",
version = "@component.version@")
)
public interface ExampleRestApiSbbLocal extends TriggerScenario, SbbLocalObject {
}
SBB class
The root SBB implements the business logic of the service.
The slee-annotations on the root SBB define the service, root SBB and a dependency on our Ping Pong SBB-Part.
In this simple example, the root SBB will write a trace message in response to triggerScenario()
.
@SleeService(
id = @ComponentId(name = "@component.name@",
vendor = "@component.vendor@",
version = "@component.version@"),
rootSbb = @RootSBB(sbb = @ComponentId(name = "example-rest-api-sbb",
vendor = "@component.vendor@",
version = "@component.version@"))
)
@SBB(
vendorExtensionID = "example-rest-api-sbb-id",
id = @ComponentId(name = "example-rest-api-sbb",
vendor = "@component.vendor@",
version = "@component.version@"),
sbbClasses = @SBBClasses(
localInterface = @SBBLocalInterface(
interfaceName = "com.exampleco.slee.rest.ExampleRestApiSbbLocal"),
abstractClass = @SBBAbstractClass(reentrant = true)
),
libraryRefs = {
@LibraryReference(library = @ComponentId(name = "example-service-sbblocal",
vendor = "@component.vendor@",
version = "@component.version@"))
}
)
@OcSBB(
vendorExtensionID = "example-rest-api-sbb-id",
sbbParts = {
@SBBPartReference(id = @ComponentId(name = "example-pingpong-api-sbbpart",
vendor = "@component.vendor@",
ersion = "@component.version@"))
},
sbbClasses = @OcSBBClasses()
)
public abstract class ExampleRestApiSbb implements javax.slee.Sbb, TriggerScenario {
-
defines the service and identifies the root SBB
-
the example SBB (the root SBB for the service)
-
the sbb-part annotation links the root SBB to our Ping Pong sbb-part
@Override
public boolean triggerScenario(String api, String scenario) {
tracer.fine("Triggering: api={}, scenario={}", api, scenario);
return true;
}
Create an sbb-part
SBB-Part class
The PingpongSbbpart
class extends the generated sbb-part superclass (PingpongApiServerSbbpart
).
Unresolved directive in <stdin> - include::/mnt/ephemeral0/workspace/product/ra/rest-api-framework/release-1.0.0/Auto/rest-api-framework-docs/rest-api-framework-public-docs/rhino-rest-api-framework-users-guide/include/PingpongSbbpart.java[tag=sbbpart-annotation]
Implement sbb-part constructor
The PingpongSbbpart
calls the superclass constructor and creates a Ping
API object.
Unresolved directive in <stdin> - include::/mnt/ephemeral0/workspace/product/ra/rest-api-framework/release-1.0.0/Auto/rest-api-framework-docs/rest-api-framework-public-docs/rhino-rest-api-framework-users-guide/include/PingpongSbbpart.java[tag=sbbpart-constructor]
Override protected methods from the generated sbb-part superclass
The generated superclass defines protected methods that our sbb-part overrides to implement the service logic of the service.
Unresolved directive in <stdin> - include::/mnt/ephemeral0/workspace/product/ra/rest-api-framework/release-1.0.0/Auto/rest-api-framework-docs/rest-api-framework-public-docs/rhino-rest-api-framework-users-guide/include/PingpongSbbpart.java[tag=override-protected-methods]
Implement handlePingRequest
by calling triggerScenario()
on the sbb-local interface,
passing the api
and scenario
values from the PingReqest
.
Understanding the Generated Code in PingPongApiServerSbbPart
The generated sbb-part superclass handles all the necessary wiring code required to access the PingPong
REST API.
-
@SBBPart
,@RATypeBindings
and @SBBPartDeployableUnit slee annotations -
SBB Part constuctor that does required JNDI lookups for the
PingPong
REST API provider -
implements event handlers for each event supported by the
PingPong
REST API -
defines protected methods that developers override to implement service logic
Unresolved directive in <stdin> - include::/mnt/ephemeral0/workspace/product/ra/rest-api-framework/release-1.0.0/Auto/rest-api-framework-docs/rest-api-framework-public-docs/rhino-rest-api-framework-users-guide/include/PingPongApiServerSbbPart.java[tag=sbbpart-annotation]
Unresolved directive in <stdin> - include::/mnt/ephemeral0/workspace/product/ra/rest-api-framework/release-1.0.0/Auto/rest-api-framework-docs/rest-api-framework-public-docs/rhino-rest-api-framework-users-guide/include/PingPongApiServerSbbPart.java[tag=sbbpart-constructor]
Unresolved directive in <stdin> - include::/mnt/ephemeral0/workspace/product/ra/rest-api-framework/release-1.0.0/Auto/rest-api-framework-docs/rest-api-framework-public-docs/rhino-rest-api-framework-users-guide/include/PingPongApiServerSbbPart.java[tag=eventhandler-request-com.exampleco.openapi.pingpong.api.PingRequest]
-
slee-annotation for the
PingRequest
event handler -
implementation of the
PingRequest
event handler -
developer overrides this method to define normal behaviour for handling a
PingRequest
-
developer overides this method to define the behaviour when handling a
PingRequest
fails.