The following is an SBB that implements a Pet Store using the Pet Store API.
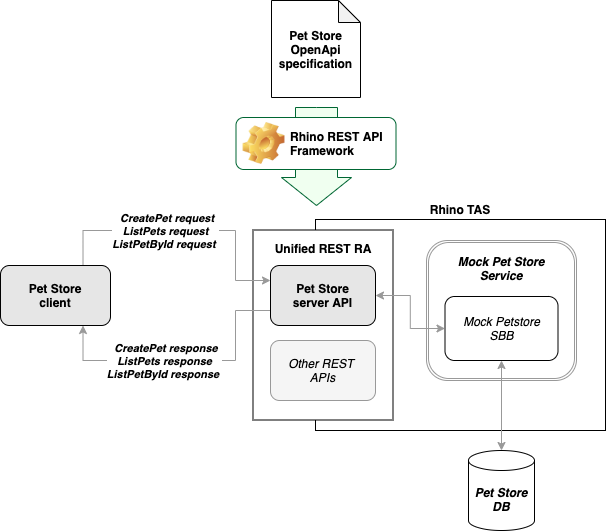
To develop the Pet Store SBB you must:
![]() |
Learn about Rhino TAS - Telecom Application Server and the JAIN SLEE (JSR 240). |
Create an SBB class and define slee-annotations
Create an abstract class that implements javax.slee.sbb
.
This class will be the root SBB
of the Pet Store service and should implement the SBB
lifecycle operations.
Define slee annotations on your abstract class that define: the Pet Store service, the root sbb and a resource adaptor type binding for the Pet Store REST API.
@SleeService(
id = @ComponentId(name = "@component.name@",
vendor = "@component.vendor@",
version = "@component.version@"),
rootSbb = @RootSBB(
sbb = @ComponentId(name = "@component.name@-sbb",
vendor = "@component.vendor@",
version = "@component.version@"))
)
@SBB(
id = @ComponentId(name = "@component.name@-sbb",
vendor = "@component.vendor@",
version = "@component.version@"),
sbbClasses = @SBBClasses(abstractClass = @SBBAbstractClass(reentrant = true)),
libraryRefs = {
@LibraryReference(
library = @ComponentId(name = "@rest-api-common.LibraryID.rest-api-common.name@",
vendor = "@rest-api-common.LibraryID.rest-api-common.vendor@",
version = "@rest-api-common.LibraryID.rest-api-common.version@"))
}
)
@RATypeBindings(
raTypeBindings = {
@RATypeBinding(
raType = @ComponentId(name = "petstore-api-server", vendor = "ExampleCo", version = "1.0"),
resourceAdaptorObjectName = MockPetstoreSbb.petstore_PROVIDER_JNDI_NAME,
resourceAdaptorEntityLink = "unified-rest-ra"
)
}
)
public abstract class MockPetstoreSbb implements javax.slee.Sbb {
Implement setSbbContext() and other SBB lifecycle operations
Rhino invokes the setSbbContext()
method on instances of your SBB abstract class.
Initialize state that can be held by the SBB object during its lifetime.
![]() |
Such state cannot be specific to an SBB entity because the SBB object might be reused during its lifetime to serve multiple SBB entities. |
The MockPetstoreSbb
creates a Tracer
, does a JNDI
lookup to get a Pet Store API provider object and finally
creates a Pets
API object.
public void setSbbContext(SbbContext context) {
sbbContext = (RhinoSbbContext) context;
tracer = (ExtendedTracer) sbbContext.getTracer(sbbContext.getSbb().getName());
tracer.finest("setSbbContext");
try {
final Context env = (Context) new InitialContext().lookup("java:comp/env");
this.apiProvider = (PetstoreApiServerProvider)
env.lookup(petstore_PROVIDER_JNDI_NAME);
}
catch (NamingException e) {
throw new RuntimeException("NamingException locating PetstoreApiServerProvider", e);
}
// get a pet store API instance
this.petsApi = apiProvider.getApiClient(
ApiConfiguration.standardConfiguration()).getPetsApi();
}
Implement event handlers
![]() |
Speed up your development by referring to the generated sbbpart superclass.
The generated event handlers in the Petstore API sbbpart superclass can be copied directly to MockPetstoreSbb .
|
On receipt of a CreatePet
request event, call handleCreatePetRequest()
.
If there is any failure then call rejectCreatePetRequest()
.
@EventMethod(
eventType = @ComponentId(name = "com.exampleco.petstore.api.CreatePetRequest",
vendor = "ExampleCo",
version = "1.0"),
initialEvent = true,
initialEventSelectVariable = IESVariableType.ActivityContext
)
public void onCreatePetRequest(CreatePetRequest request,
ActivityContextInterface aci,
EventContext eventContext)
{
tracer.finest("Received: {}", request);
try {
handleCreatePetRequest(request, aci);
}
catch(IOException e) {
tracer.finest("Failed to handle: {}", request, e);
rejectCreatePetRequest(request, aci);
}
}
Process a CreatePet
request by updating the Pets DB.
If updating the DB is successful, then send a success response (request.create_204_SuccessResponse()
).
Otherwise send a 501
error response.
private void handleCreatePetRequest(CreatePetRequest request,
ActivityContextInterface aci) throws IOException
{
if (addPet(request.getPet())) {
tracer.finest("Added pet: {}", request.getPet());
sendResponse(request.create_204_SuccessResponse(), aci);
}
else {
final RestResponseBuilder errorResponse = request.createDefaultResponse(
501,"application/json",
new Error().code(501)
.message("Pet already exists with id: " + request.getPet().getId()));
sendResponse(errorResponse, aci);
}
}
Reject a CreatePet
request, by sending a 501
error response.
private void rejectCreatePetRequest(CreatePetRequest request, ActivityContextInterface aci) {
final RestResponseBuilder errorResponse = request.createDefaultResponse(
501,"application/json",
new Error().code(501).message("Failed to create pet: " + request.getPet()));
sendResponse(errorResponse, aci);
}
private void sendResponse(RestResponseBuilder response, ActivityContextInterface aci) {
try { petsApi.sendResponse(response, aci); }
catch (IOException e) {
tracer.finest("Failed to send response. Api = {} , OperationId = {}",
response.getApi(), response.getOperationId(), e);
}
}
Implementation of the ListPetsRequest
and ShowPetByIdRequest
event handlers follows the same pattern.
@EventMethod(
eventType = @ComponentId(name = "com.exampleco.petstore.api.ListPetsRequest",
vendor = "ExampleCo",
version = "1.0"),
initialEvent = true,
initialEventSelectVariable = IESVariableType.ActivityContext
)
public void onListPetsRequest(ListPetsRequest request,
ActivityContextInterface aci,
EventContext eventContext)
{
tracer.finest("Received: {}", request);
try {
handleListPetsRequest(request, aci);
}
catch(IOException e) {
tracer.finest("Failed to handle: {}", request, e);
rejectListPetsRequest(request, aci);
}
}
@EventMethod(
eventType = @ComponentId(name = "com.exampleco.petstore.api.ShowPetByIdRequest",
vendor = "ExampleCo",
version = "1.0"),
initialEvent = true,
initialEventSelectVariable = IESVariableType.ActivityContext
)
public void onShowPetByIdRequest(ShowPetByIdRequest request,
ActivityContextInterface aci,
EventContext eventContext)
{
tracer.finest("Received: {}", request);
try {
handleShowPetByIdRequest(request, aci);
}
catch(IOException e) {
tracer.finest("Failed to handle: {}", request, e);
rejectShowPetByIdRequest(request, aci);
}
}