Before the SIS can execute a composition, it must first decide which composition to run. Triggers use rules and conditional logic to define how the SIS selects a composition when it receives an initial request. Multiple triggers may be active at the same time, and the SIS will evaluate them sequentially in order of their priority.
The SIS executes the first composition that a trigger successfully returns (using the initial request as input). If evaluating all triggers returns no compositions, the SIS considers the initial request invalid and replies with a failure response.
What’s in a trigger?
A trigger contains the following information:
-
an optional description
-
name, vendor, and version identifiers
-
a priority, which the SIS uses to determine the order in which it evaluates triggers — ranging from 127 (highest) to -128 (lowest)
-
references to any required macros, compositions, or services
-
a condition expression
-
rules for selecting a composition: zero or more selectors and optional terminate clauses.
Evaluating triggers
The following pseudo-code describes how the SIS evaluates triggers when processing an initial request it receives from the network:
Set T = set of all active triggers
while T is not empty
remove highest priority trigger from T
if trigger condition evaluates to true
// process selectors
for each selector in the trigger
invoke selector
if selector returns a valid composition
return selected composition as trigger result
endif
if selector returns a terminate result
// a Service Composition Selection event returned a "terminate" result
return terminate result as trigger result
endif
endfor
// process terminate clause
if selector contains a terminate clause
return terminate clause as trigger result
endif
endif
endwhile
// no active triggers were satisfied
return SIS generic failure result as trigger result
![]() |
About the generic failure result
The SIS defines the generic failure result as a last-ditch attempt to return a trigger selection result. OpenCloud highly recommends a set of triggers that provide their own failure handling (rather than relying on the SIS generic failure result) — for example, by using a trigger such as the generic failure handler. The SIS generic failure result for |
Trigger conditions
Whether the SIS tries to use a trigger depends on its condition:
-
If the condition evaluates to
true
, the SIS evaluates the selectors and/or failure clause. -
If the condition evaluates to
false
, the SIS disregards the trigger (for that initial request).
The following example has a trigger condition that checks for INVITE
messages that correspond to originating
access. The trigger condition checks to see that the SIP method is INVITE
and also checks for the presence of an orig
parameter in the Route
uri. If the condition is true (<match>
in the diagram), then the SIS will execute the trigger body to select a composition. If the condition is not true, then the SIS will move on to the next trigger (<next-trigger>
in the diagram).
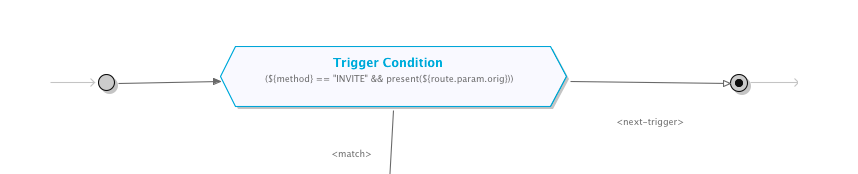
Trigger syntax schema
The base XML schema sis-trigger-1.6.xsd defines the basic structure of a trigger. SIS variants extend this base schema with protocol-specific properties.
IN Triggers
Triggers for IN protocols may use the XML schema in-sis-trigger-1.6.xsd to extend the generic schema for SIS triggers. The corresponding schema namespaces used by such triggers is shown below:
<trigger xmlns="http://www.opencloud.com/SIS/Trigger"
xmlns:in="http://www.opencloud.com/SIS/Trigger/IN"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://www.opencloud.com/SIS/Trigger/IN in-sis-trigger-1.6.xsd
http://www.opencloud.com/SIS/Trigger sis-trigger-1.6.xsd">
SIP Triggers
Triggers for SIP may use the XML schema sip-sis-trigger-1.6.xsd to extend the generic schema for SIS triggers. The corresponding schema namespaces used by such triggers is shown below:
<trigger xmlns="http://www.opencloud.com/SIS/Trigger"
xmlns:sip="http://www.opencloud.com/SIS/Trigger/SIP"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://www.opencloud.com/SIS/Trigger/SIP sip-sis-trigger-1.6.xsd
http://www.opencloud.com/SIS/Trigger sis-trigger-1.6.xsd">
Sample trigger: IN trigger-event processing
The following trigger processes originating IN initial requests first by normalising the A
party numbers, and then by using the normalised values in a profile selection based on address subscription.
If that fails, it tries to select a composition using a service key subscription profile.
If that too fails, the trigger returns with a User Error
failure response that indicates a missing customer record.
IN example: VIA diagram
In this IN example, the SIS will evaluate the trigger body if it’s part of an originating trigger, and the calling party number can be normalised. If the condition applies, it then try to locate a composition:
-
by searching the
address-subscriptions
table for the normalisedredirecting-party-id
-
by searching the
address-subscriptions
table for the normalisedcalling-party-number
-
by searching the
service-key-subscriptions
table for theservice-key
.
If none of that works, it terminates with a missing customer record user error, recorded in statistics as a failure.
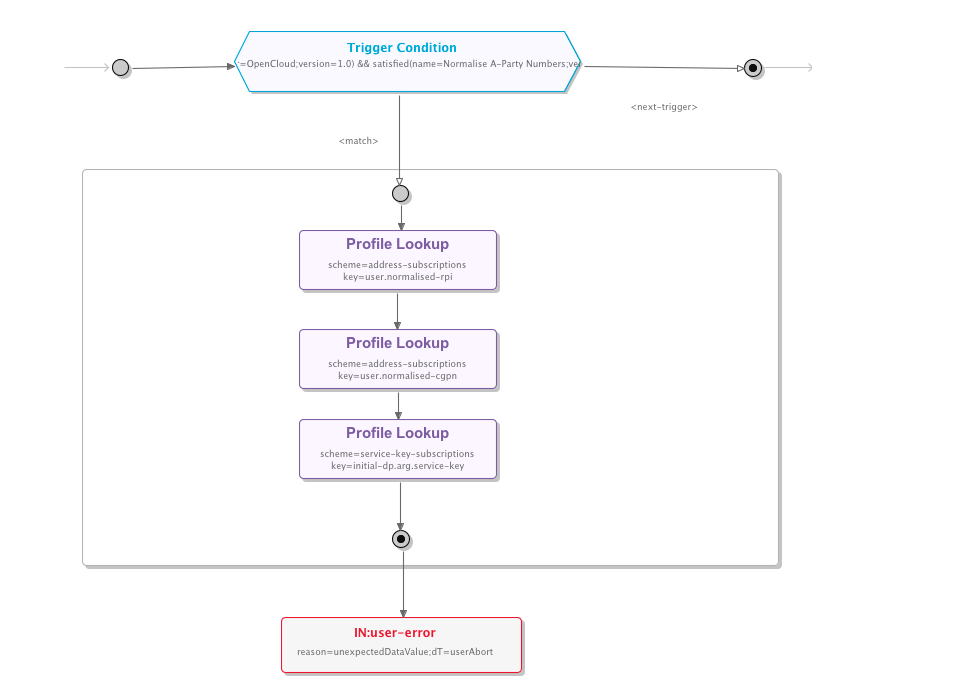
IN example: trigger XML
<trigger xmlns="http://www.opencloud.com/SIS/Trigger"
xmlns:in="http://www.opencloud.com/SIS/Trigger/IN"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://www.opencloud.com/SIS/Trigger/IN in-sis-trigger-1.6.xsd
http://www.opencloud.com/SIS/Trigger sis-trigger-1.6.xsd">
<description>
Handle O triggers by looking for a service composition using the
RedirectingPartyID, CallingPartyNumber, and ServiceKey (in that order)
</description>
<trigger-name>O-Trigger Handling</trigger-name>
<trigger-vendor>OpenCloud</trigger-vendor>
<trigger-version>1.0</trigger-version>
<trigger-priority>99</trigger-priority>
<macro-ref>
<macro-name>O-Trigger</macro-name>
<macro-vendor>OpenCloud</macro-vendor>
<macro-version>1.0</macro-version>
<macro-alias>O-Trigger</macro-alias>
</macro-ref>
<macro-ref>
<macro-name>Normalise A-Party Numbers</macro-name>
<macro-vendor>OpenCloud</macro-vendor>
<macro-version>1.0</macro-version>
<macro-alias>Normalise A-Party Numbers</macro-alias>
</macro-ref>
<on-condition>
<and>
<!-- if the IDP is an O trigger ... -->
<macro-satisfied macro="O-Trigger"/>
<!-- ...normalise the A party numbers for the profile lookup -->
<macro-satisfied macro="Normalise A-Party Numbers"/>
</and>
</on-condition>
<!-- ... try selecting on the redirecting party id, then calling party number, then service key ... -->
<select>
<profile-lookup scheme="address-subscriptions" key="${user.normalised-rpi}"/>
</select>
<select>
<profile-lookup scheme="address-subscriptions" key="${user.normalised-cgpn}"/>
</select>
<select>
<profile-lookup scheme="service-key-subscriptions" key="${initial-dp.arg.service-key}"/>
</select>
<!-- if none of that worked, fail with a missing customer record user error -->
<in:terminate record-as-reject="true">
<in:user-error reason="missing-customer-record" dialog-termination="user-abort"/>
</in:terminate>
</trigger>
Sample trigger: SIP trigger-event processing
The following trigger matches initial SIP INVITE
requests that have the "orig" (originating) parameter set in the Route header. If a request matches, the trigger uses the From header’s URI to select the composition. If the trigger does not find a matching composition, it returns a 404 error response to the client.
SIP example: VIA diagram
In this SIP example, the SIS will evaluate the trigger body if it has received an originating INVITE request. If the condition applies, it tries to locate a composition by searching the originating-address
table for the From header’s URI
. If that does not work, it fails with a SIP 404
error response.
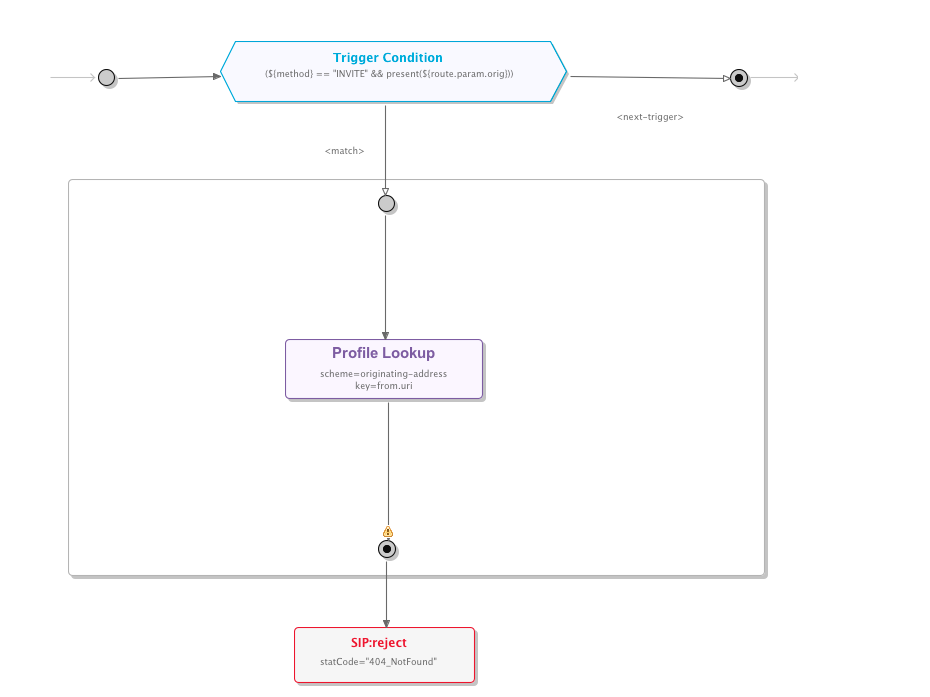
SIP example: trigger XML
<trigger xmlns="http://www.opencloud.com/SIS/Trigger"
xmlns:sip="http://www.opencloud.com/SIS/Trigger/SIP"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://www.opencloud.com/SIS/Trigger/SIP sip-sis-trigger-1.6.xsd
http://www.opencloud.com/SIS/Trigger sis-trigger-1.6.xsd">
<description>
Runs the originating composition if an originating INVITE request is received.
</description>
<trigger-name>Originating</trigger-name>
<trigger-vendor>OpenCloud</trigger-vendor>
<trigger-version>1.0</trigger-version>
<trigger-priority>20</trigger-priority>
<!-- Trigger on INVITE request with "orig" Route header parameter. -->
<on-condition>
<and>
<equal a="${method}" b="INVITE"/>
<present variable="${route.param.orig}"/>
</and>
</on-condition>
<select>
<!-- SIS will lookup the subscriber address in its subscription profiles,
and select the originating composition, if available -->
<profile-lookup scheme="originating-address" key="${from.uri}"/>
</select>
<sip:terminate>
<sip:reject status-code="404"/>
</sip:terminate>
</trigger>
Sample trigger: generic failure handler
The following trigger illustrates a user-defined generic failure handler for IN. This trigger has the lowest possible priority and a condition that always evaluates to true
. If ever evaluated, this trigger causes a User Error
with cause unexpected data value
returned as the trigger result.
Sample generic failure handler: trigger XML
<trigger xmlns="http://www.opencloud.com/SIS/Trigger"
xmlns:in="http://www.opencloud.com/SIS/Trigger/IN"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://www.opencloud.com/SIS/Trigger/IN in-sis-trigger-1.6.xsd
http://www.opencloud.com/SIS/Trigger sis-trigger-1.6.xsd">
<description>Generic catch-all failure handler</description>
<trigger-name>IN Generic Failure Handler</trigger-name>
<trigger-vendor>OpenCloud</trigger-vendor>
<trigger-version>1.0</trigger-version>
<trigger-priority>-128</trigger-priority>
<on-condition>
<true/>
</on-condition>
<in:terminate record-as-reject="true">
<in:user-error reason="unexpected-data-value" dialog-termination="user-abort"/>
</in:terminate>
</trigger>