CGIN adopts some features and design patterns from INCC. One important feature of the INCC APIs that has been preserved is the idea of abstracting the common features of call control APIs into a common API — this was the INCC (IN Call Control) API.
Concrete call control protocols (such as INAP and CAP) were supported with APIs that extended INCC (for example CAPv2InitialDPReq
is a sub-type of InitialDPReq
). The INCC API (and extensions for each concrete protocol) were all hand-crafted. CGIN APIs, on the other hand, are almost entirely code-generated.
This page presents the CGIN family of APIs and also discusses some important differences in the design patterns adopted for the CGIN APIs.
CGIN family of APIs
CGIN provides a suite of APIs for INAP, CAP, and MAP. The diagram below shows the set of APIs provided in the CGIN Connectivity Pack.
Call control protocol APIs are defined as a hierarchy where each protocol API is defined as an extension of another protocol API. For example CAP v4 extends CAP v3, which extends CAP v2, which extends CAP v1.
At the root of the hierarchy is the CGIN Call Control (CGIN CC) API. CGIN CC is a synthetic call control protocol that is the intersection of ETSI INAP CS1 and CAP v4. Call control applications can be written in terms of the CGIN CC API and may be largely independent of the actual signaling protocols used.
![]() |
The CGIN Call Control API is also generated by the CGIN toolchain. CGIN CC has its own ASN.1 description, which is why we refer to it as a synthetic protocol. |
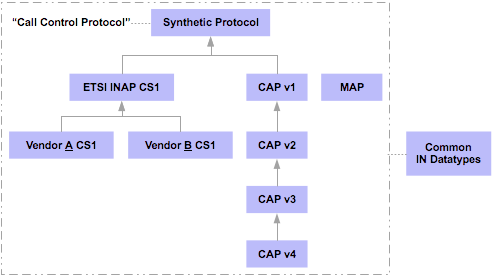
-
Call Control API — API for the synthetic protocol that is the intersection of INAP and CAP.
The CGIN Call Control API plays the same role as the core/common classes of the deprecated INCC (IN Call Control) API. -
INAP APIs — A family of INAP APIs that are based on a common definition of ETSI INAP CS1
-
ETSI INAP CS1 (ETS 300 374-1: September 1994)
-
Please contact OpenCloud for information pertaining to INAP variants supported
-
-
CAP APIs — A family of CAP APIs that are based on the definition of CAP v1
-
CAP v.1 (ETSI TS 101 046 / GSM 09.78 V5.7.0 Release 1996)
-
CAP v.2 (ETSI TS 101 046 / GSM 09.78 V7.1.0 Release 1998)
-
CAP v.3 (3GPP TS 29.078 V4.8.0 Release 4)
-
CAP v.4 (3GPP TS 29.078 v9.1.1 Release 9)
-
-
MAP API — An API for GSM MAP.
-
Mobile Application Part (MAP) (3GPP TS 29.002 v10.0.0 Release 10)
-
-
IN Datatypes API — A library of classes to represent types for which their ASN.1 specification is
OCTET STRING
, and for which standards define the layout of bit-fields within the octet string (for exampleCalledPartyNumber
andISDNSubaddress
).
![]() |
Learn more…
See: CGIN APIs for javadoc of all APIs in the CGIN family. |
An API design pattern for SS7 APIs
An updated pattern for our SS7 APIs has been introduced as a part of CGIN. We decided to make this change based on our own experiences using our INCC APIs and on our customers' feedback. There are several reasons why we did this:
-
There are some common use cases for programming with our SS7 APIs that were harder to implement than we liked. For example it was difficult to copy a message from one dialog to another.
-
We noticed some common errors programmers were making using our APIs. These were related to rules imposed on the programmer by the SLEE programming model that programmers were not following.
-
We can guarantee all CGIN APIs are mutually consistent, with consistent naming schemes, by using code-generation techniques. We could not provide this guarantee previously, as INCC APIs were hand-crafted.
CGIN APIs
The CGIN APIs represent an SS7 message as a POJO (Plain Old Java Object). This SS7 message POJO is only responsible for encapsulating the fields of the SS7 message it represents. Applications can therefore safely store messages in CMP fields.
The CGIN CC InitialDP, for example, is represented by the CCInitialDPArg
class.
// A POJO that represents an InitialDP
public class CCInitialDPArg extends AbstractFieldsObject {
public CalledPartyNumber getCalledPartyNumber() { ... }
public CallingPartyNumber getCallingPartyNumber() { ... }
// + other methods for accessing fields of the InitialDP
}
SLEE events are represented as a separate class with an associated message POJO.
The CGIN CC InitialDP request, for example, is shown below. The CCInitialDPRequestEvent
extends from the OperationInvokeEvent
generic class, with a type parameter value of CCInitialDPArg
. CCInitialDPArg
is the POJO class that represents the InitialDP message itself.
/**
* Base class for all operation-specific invoke event classes.
* Individual protocols subclass this event for each operation they support.
* Each operation defines its own event type.
*/
public class OperationInvokeEvent<ArgType> extends OperationEvent {
public ArgType getArgument() { return argument; }
// ...
}
// ...
public class CCInitialDPRequestEvent extends OperationInvokeEvent<CCInitialDPArg> {
// ...
}
INCC APIs
In the INCC APIs, each SS7 message was represented with a Java interface. This interface fulfilled two roles: 1) it represented the event object that the resource adaptor submitted to the SLEE and 2) it encapsulated all the fields of the SS7 message it represented.
The INCC InitialDP request, for example, is shown below. It is a Java interface that represents both the InitialDP request event and the fields of the InitialDP message.
/**
* This interface defines the IN Call Control InitialDP request message that
* may be sent to the network.
*/
public interface InitialDPReq extends Request {
public CalledPartyNumber getCalledPartyNumber();
public CallingPartyNumber getCallingPartyNumber();
// + other operations for accessing fields of the InitialDP
}
![]() |
Restrictions imposed by the SLEE programming model
The SLEE programming model imposes some restrictions on what a SLEE application can do with an event. An important one that programmers often misunderstand (or simply forget!) is that event objects should not be stored by the service in CMPs (or by using any other method) for use in future transactions. This is simply because the event is owned by the resource adaptor and the SLEE has no knowledge of how the event object has been implemented (and therefore cannot make any assumptions in terms of persistence and so on). |